It starts with a single line.
A line that could make or break your app's success.
We're talking about stock charts in Flutter - the feature that can transform your financial app from mediocre to magnificent.
But creating compelling stock charts isn't just about plotting points on a graph.
It's about telling a story with data, a story that can empower users to make informed financial decisions.
And yet, many developers struggle to create charts that are both beautiful and functional.
They don't realize that with the right approach, Flutter can produce charts that rival even the most sophisticated desktop trading platforms.
Are you ready to unlock the secrets of creating stunning stock charts that will make your app stand out in the crowded fintech market?
Then let's dive into the world of Flutter stock charts and discover how you can become a master of financial data visualization...
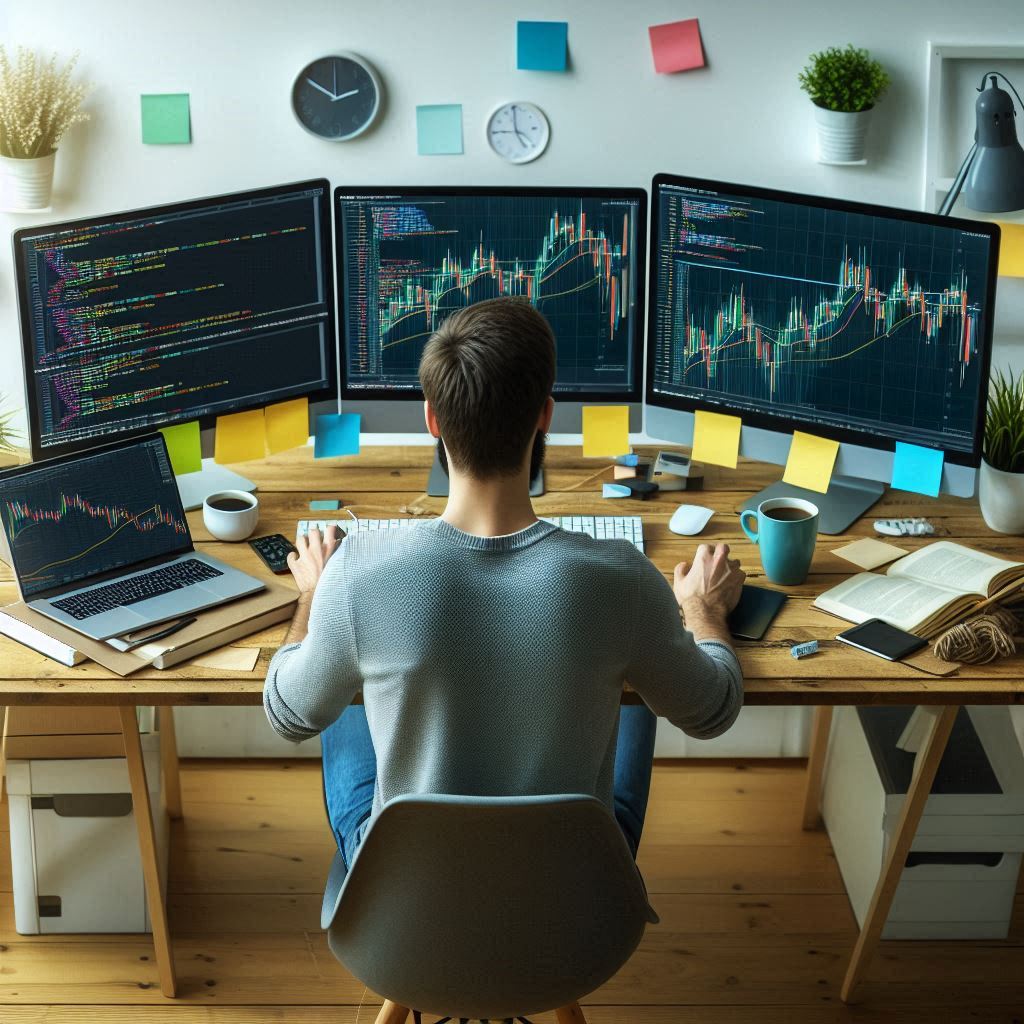
1. Introduction: The Power of Data Visualization in Mobile Apps
In a world drowning in data, visualization is the life raft that keeps users afloat. Nowhere is this more true than in financial apps, where complex data needs to be understood at a glance. Stock charts are not just pretty pictures; they're the interface between raw data and human decision-making.
But here's the kicker: creating effective stock charts is an art as much as it is a science. It's about finding the perfect balance between information density and clarity, between beauty and functionality. And that's where Flutter comes in.
2. Flutter: The Swiss Army Knife for Stock Chart Creation
Flutter isn't just another cross-platform framework; it's a game-changer for financial app development. Here's why:
- Performance: Flutter's compiled native code runs blazingly fast, essential for real-time stock data.
- Customizability: With Flutter, every pixel is under your control, allowing for truly unique chart designs.
- Rich ecosystem: A plethora of packages specifically designed for financial charting.
- Cross-platform: Build once, deploy everywhere - from iOS to Android, web to desktop.
3. The Holy Trinity of Flutter Chart Packages
3.1. fl_chart: The Jack of All Trades
Fl_chart is like the Swiss Army knife of Flutter charting. It's versatile, relatively easy to use, and can create a wide range of chart types. Perfect for those who want a one-stop solution for all their charting needs.
3.2. syncfusion_flutter_charts: The Professional's Choice
If fl_chart is a Swiss Army knife, syncfusion_flutter_charts is a surgical scalpel. It offers precision, a wide range of financial chart types, and performance that can handle large datasets. The downside? It comes with a price tag for commercial use.
3.3. charts_flutter: Google's Offering
Straight from the creators of Flutter, charts_flutter offers solid performance and integration. It's not as feature-rich as the others, but its simplicity can be a strength for basic charting needs.
4. From Zero to Hero: Creating Your First Flutter Stock Chart
4.1. Setting Up Your Flutter Project
Before we dive into the code, let's set up our Flutter project. Open your terminal and run:
flutter create flutter_stock_chart
cd flutter_stock_chart
4.2. Adding Dependencies
For this tutorial, we'll use fl_chart. Add it to your pubspec.yaml:
dependencies:
flutter:
sdk: flutter
fl_chart: ^0.55.0
4.3. Preparing the Data
Let's create a simple data model for our stock prices:
class StockPrice {
final DateTime date;
final double price;
StockPrice(this.date, this.price);
}
4.4. Creating the Basic Chart
Now, let's create a basic line chart:
import 'package:fl_chart/fl_chart.dart';
class StockChart extends StatelessWidget {
final List<StockPrice> prices;
StockChart({required this.prices});
@override
Widget build(BuildContext context) {
return LineChart(
LineChartData(
lineBarsData: [
LineChartBarData(
spots: prices.map((price) => FlSpot(
price.date.millisecondsSinceEpoch.toDouble(),
price.price,
)).toList(),
isCurved: true,
color: Colors.blue,
),
],
),
);
}
}
4.5. Styling Your Chart
A bare chart is functional, but not very appealing. Let's add some style:
LineChartData(
gridData: FlGridData(show: false),
titlesData: FlTitlesData(show: false),
borderData: FlBorderData(show: false),
lineBarsData: [
LineChartBarData(
spots: prices.map((price) => FlSpot(
price.date.millisecondsSinceEpoch.toDouble(),
price.price,
)).toList(),
isCurved: true,
color: Colors.blue,
barWidth: 3,
isStrokeCapRound: true,
dotData: FlDotData(show: false),
belowBarData: BarAreaData(
show: true,
color: Colors.blue.withOpacity(0.3),
),
),
],
)
5. Advanced Techniques: Taking Your Charts to the Next Level
5.1. Adding Interactivity
Static charts are so last decade. Let's add some interactivity:
LineChartData(
lineTouchData: LineTouchData(
touchTooltipData: LineTouchTooltipData(
tooltipBgColor: Colors.blueAccent,
getTooltipItems: (List<LineBarSpot> touchedBarSpots) {
return touchedBarSpots.map((barSpot) {
final flSpot = barSpot;
return LineTooltipItem(
'${flSpot.y}',
const TextStyle(color: Colors.white),
);
}).toList();
},
),
handleBuiltInTouches: true,
),
// ... rest of your chart data
)
5.2. Implementing Technical Indicators
For the true finance geeks, let's add a simple moving average:
List<FlSpot> calculateSMA(List<StockPrice> prices, int period) {
List<FlSpot> smaData = [];
for (int i = period - 1; i < prices.length; i++) {
double sum = 0;
for (int j = i - period + 1; j <= i; j++) {
sum += prices[j].price;
}
double sma = sum / period;
smaData.add(FlSpot(prices[i].date.millisecondsSinceEpoch.toDouble(), sma));
}
return smaData;
}
// Then add this to your chart data:
LineChartBarData(
spots: calculateSMA(prices, 20),
isCurved: true,
color: Colors.red,
barWidth: 2,
)
5.3. Animating Your Chart
Let's bring your chart to life with some smooth animations:
class _StockChartState extends State<StockChart> {
bool isShowingMainData = true;
@override
Widget build(BuildContext context) {
return AspectRatio(
aspectRatio: 1.70,
child: LineChart(
isShowingMainData ? mainData() : secondaryData(),
swapAnimationDuration: const Duration(milliseconds: 250),
),
);
}
LineChartData mainData() {
// Your main chart data
}
LineChartData secondaryData() {
// Your secondary chart data (e.g., different time frame)
}
}
6. Integrating Real-Time Stock Data
Now that we have a beautiful chart, let's feed it with real data. Here's a simple way to integrate with a stock API:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<List<StockPrice>> fetchStockData() async {
final response = await http.get(Uri.parse('https://api.example.com/stock/AAPL'));
if (response.statusCode == 200) {
List<dynamic> pricesJson = jsonDecode(response.body);
return pricesJson.map((json) => StockPrice(
DateTime.parse(json['date']),
json['price'].toDouble(),
)).toList();
} else {
throw Exception('Failed to load stock data');
}
}
7. Performance Optimization: Handling Large Datasets
When dealing with years of stock data, performance can become an issue. Here are some tips:
- Use data decimation techniques to reduce the number of points displayed
- Implement lazy loading to fetch data as the user scrolls
- Use efficient data structures like LinkedList for fast insertions and deletions
8. Best Practices and Common Pitfalls
As you venture deeper into the world of Flutter stock charts, keep these tips in mind:
- Always handle edge cases (empty data, extreme values)
- Provide clear labels and legends
- Use color wisely - red and green have specific meanings in finance
- Test your charts on different screen sizes and orientations
- Don't forget about accessibility - provide alternative text descriptions for your charts
9. Flutter vs Native: The Charting Showdown
How do Flutter stock charts stack up against native solutions? Here's a quick comparison:
Feature | Flutter | Native (iOS/Android) |
---|---|---|
Performance | Excellent | Slightly better |
Development Speed | Fast | Slower (two codebases) |
Customization | High | Very High |
Learning Curve | Moderate | Steep (two platforms) |
10. The Future of Financial Visualization in Flutter
The world of Flutter and financial visualization is evolving rapidly. Keep an eye on these trends:
- Machine learning integration for predictive charting
- AR/VR visualizations for immersive financial analysis
- Increased focus on real-time, streaming data visualization
- More sophisticated, finance-specific charting packages
11. Conclusion: Your Next Steps in Mastering Flutter Stock Charts
Congratulations! You've taken your first steps into the exciting world of Flutter stock charts. But this is just the beginning. Here's what you can do next:
- Experiment with different chart types (candlestick, OHLC)
- Dive deeper into technical indicators
- Explore more advanced packages like syncfusion_flutter_charts
- Build a complete trading app using your new charting skills
Remember, creating effective stock charts is as much an art as it is a science. Keep practicing, keep learning, and soon you'll be creating financial visualizations that not only inform but inspire.
Now, go forth and chart your path to Flutter mastery!